Table of Contents
Memory Segment of the code
Introduction
Memory in Embedded Systems is categorized as primary and secondary memory, each having its role to play in the working of the unit collectively.
In general, hex code that is flashed onto the system is stored in an organized manner. The compartmentalization is as below. This is commonly referred to as the memory layout of a program in an Embedded System. Let’s understand them in detail. (Please Note: This is applicable to general c code execution by the CPU; here it is in the context of an embedded system).
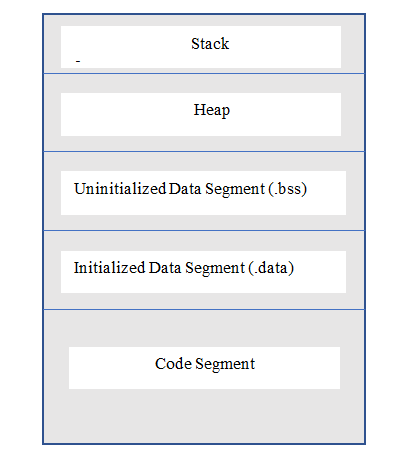
Typically, the embedded C code (singular/plural) undergoes a series of processes before it can be flashed onto the system flash and is critical in the formulation of an embedded image; it thus makes sense to have a high-level understanding of this.
CONCEPT 1
This is pictorially represented below
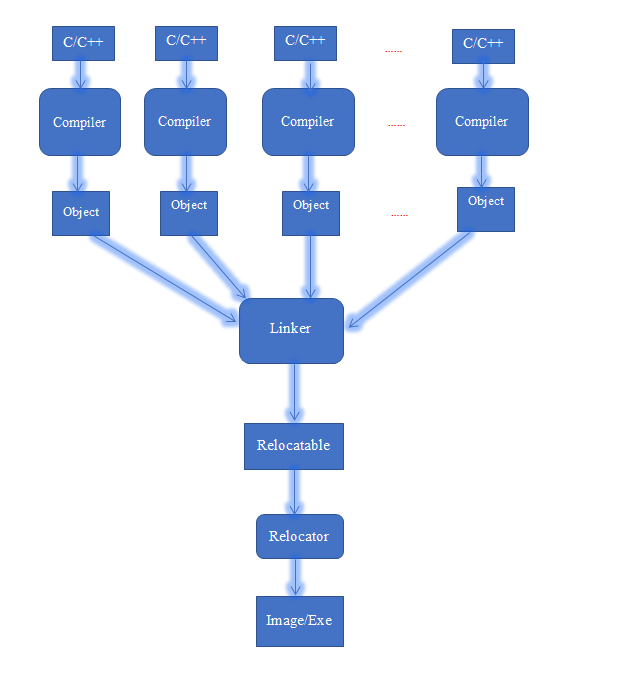
The final image is what is flashed to the embedded device. More on this later
CONCEPT 2
Once the target is flashed with this image; most often, this goes into the flash memory. This is the non-volatile memory storage unit where the code is referenced from.
Depending on the make of the processor and the way the PCB is designed; the boot up of the core slightly varies. In the sense; the MSP or the Main Stack Pointer, points to the address space of either the flash; the SRAM or the system memory (Boot Loader).
This further invokes the system reset handler which takes care of general hardware initialization and then call the software init which is commonly called as main ().
The booting process will be explained in detail on a separate write-up.
For the sake of keeping this write up to the point; Let us assume the flash memory is referenced by the MSP and how data is segmented internally; is the topic of discussion today.
Code Segment
The code Segment is the space where the program code is stored and it is further explained below
- System execution starts by referencing to the base address of this space after the boot loader does its job (This will be further explained below).
- This is typically stored in the non-volatile memory for obvious reasons i.e., secondary memory and moved to different sections of the RAM as and when it is required.
- More often, memory to store the application code resides in the flash memory; and in bigger application EEPROM’s are used.
- Constants are stored in the Code Segment of the Embedded Memory Architecture. The use of keyword const facilitates that.
Note: It is important to understand the use of const; it is imperative to use const while having data or a data structure that remains unchanged throughout the execution of the program. This prevents unnecessary RAM consumption at run time. A good coding practice if you will 🙂
Initialized Data Segment (.Data)
This segment stores the following type of storage classes
- Global variables initialized by user.
- Local Static variables initialized to any value but 0.
Note: This section can be modified at run time and hence is further categorized into initialized read-only and initialized read-write.
Un-Initialized Data Segment (.BSS)
This segment stores the following type of storage classes
- Uninitialized user variables.
- Variables that are assigned to 0.
This can be explained with the help of the following example.
#incude <stdio.h>
Static int gummyBear = 230; /* Global Variable: This is stored in the initialized data segment .Data */
Char Introduction[] = “ Hope you are enjoying this so far “ /* Global Variable: This is stored in the initialized data segment .Data */
Char x; /* Global Variable stored in the BSS Segment */
Void main()
{
Static int buzz = 113; /* Static Local Variable stored in the Initialized Data Segment */
Static int xyz ; /* Uninitialized Static Variable stored in the BSS Segment */
.
.
. /* Hope this puts things to perspective */
Return 0;
}
Heap
Dynamic memory allocation takes place in this region of the segment. Typical C constructs such as malloc(), realloc() etc employ this storage space. A heap typically grows upwards, trying to accommodate more run time memory allocations. The area is shared across all libraries and modules.
The typical faults at this region are memory leaks. If the segment is not properly utilized; memory leaks are the result and the programmer should be wary of this.
It is a good practice to free the variable once it’s purpose is fulfilled.
Ex
Char *a = (char*) malloc ( sizeof(char)); /* Memory stored in Heap */
Free (a);
Stack
The stack is a defined space where the local variables are stored; in addition other function references if any ; the base address of that function is brought into the stack. Once the execution of the function ends and it returns to the calling point; the address is popped from the stack. Too confused? Consider this,
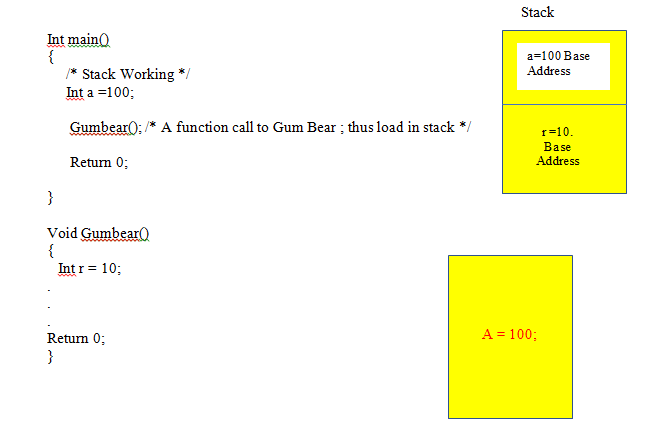
Please note a = 100; remains until the return statement is reached. Note; all recursive calls and additional branched functions will be stored in the stack. Developers need to be prudent in its usage.
The stack grows downwards unlike the Heap and there are good chances of having an overflow or other memory corruptions if proper care is not taken.
Environment
The segment which stores the CLI commands/ Inputs and other environment concerning your code is stored in this memory location.
This summarizes the memory segmentation concepts.
Note: The featured image does not belong to me. Credit goes to the ower alone!
As always thanks for reading.
Crisp and clear
I got some more clarity in memory chapter. Very helpful for embedded engineers.
Articulated in such, even a rookie get it point-blank
Articulated in such, even a rookie gets it point-blank
Simple and crisp!
Good
Good grasp of concepts mate!
Amazing article, keep writing!!